Encapsulation, Abstraction, and Composition in JavaScript
- ken quiggins
- Jun 12, 2023
- 3 min read
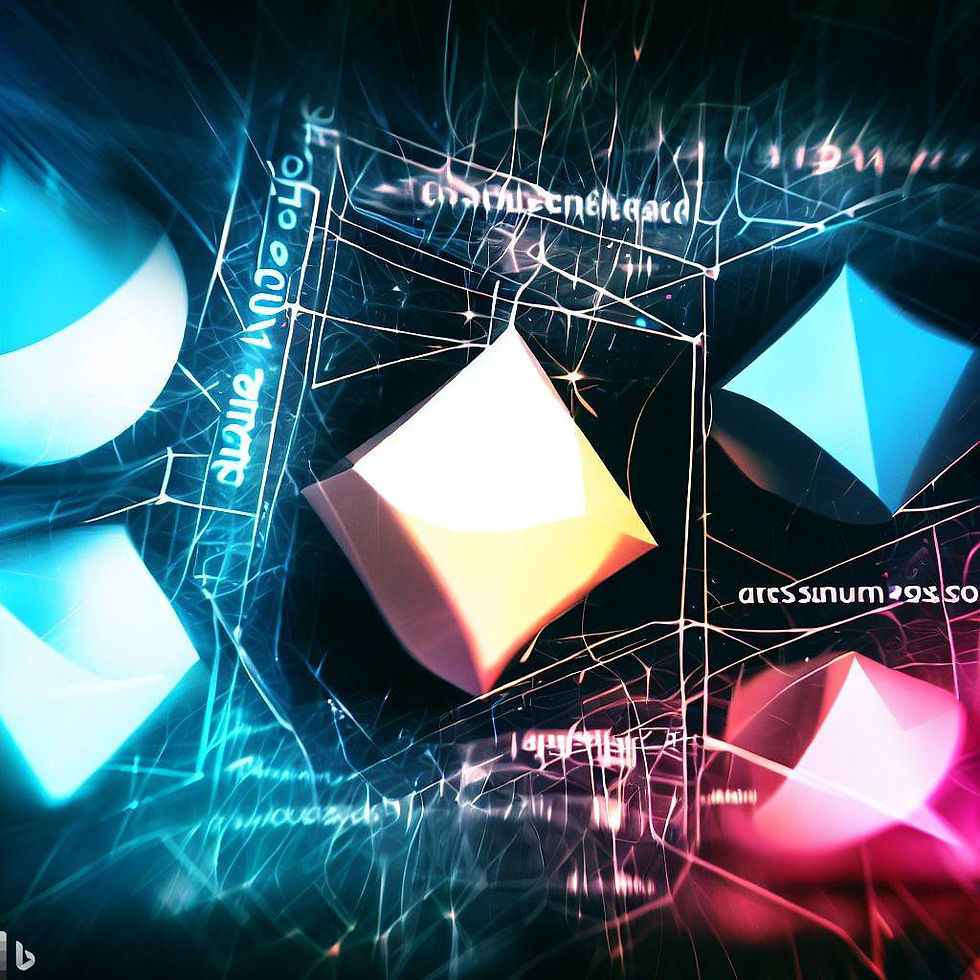
When it comes to writing clean and maintainable code, three important principles in object-oriented programming (OOP) are encapsulation, abstraction, and composition. These concepts help in organizing code, reducing complexity, and improving code reusability. In this blog post, we will delve into each of these principles and provide detailed examples of their implementation in JavaScript.
Encapsulation
Encapsulation is the practice of bundling related properties and methods into a single unit, known as a class or an object. It provides a way to control access to the internal state of an object, ensuring that it can only be modified through designated methods. This concept promotes information hiding and protects the integrity of the object's data.
Here's an example that demonstrates encapsulation in JavaScript:
class BankAccount {
constructor(accountNumber, balance) {
this._accountNumber = accountNumber;
this._balance = balance;
}
getAccountNumber() {
return this._accountNumber;
}
deposit(amount) {
this._balance += amount;
}
withdraw(amount) {
if (amount <= this._balance) {
this._balance -= amount;
} else {
console.log("Insufficient funds");
}
}
}
In this example, the BankAccount class encapsulates the accountNumber and balance properties, providing public methods like getAccountNumber(), deposit(), and withdraw() to interact with them. The properties are prefixed with an underscore (_) conventionally indicating that they are intended to be private.
By encapsulating the internal state of the BankAccount object, we control access to its properties and ensure that they are modified in a controlled manner.
Abstraction
Abstraction is the process of representing complex real-world entities in a simplified manner, focusing only on the essential details. It allows developers to work with high-level concepts without worrying about the low-level implementation details. Abstraction helps in managing complexity, improving code maintainability, and promoting code reuse.
In JavaScript, abstraction can be achieved by defining classes that provide a simplified interface while hiding the underlying implementation details.
Consider the following example:
class Shape {
constructor(color) {
this._color = color;
}
getColor() {
return this._color;
}
calculateArea() {
// Abstract method to be implemented by subclasses
}
}
class Circle extends Shape {
constructor(color, radius) {
super(color);
this._radius = radius;
}
calculateArea() {
return Math.PI * Math.pow(this._radius, 2);
}
}
class Rectangle extends Shape {
constructor(color, width, height) {
super(color);
this._width = width;
this._height = height;
}
calculateArea() {
return this._width * this._height;
}
}
In this example, the Shape class represents a general shape with a color property and an abstract method calculateArea(). Subclasses like Circle and Rectangle extend the Shape class and provide their own implementations of the calculateArea() method. The details of the area calculation are abstracted away, allowing the user to work with shapes without worrying about the specific formulas.
By using abstraction, we can work with high-level concepts and interact with objects using a simplified interface, leading to cleaner and more maintainable code.
Composition
Composition is a technique that allows objects to be constructed by combining simpler objects or components. It enables code reuse by assembling objects with different behaviors and functionalities. Instead of relying on inheritance, composition focuses on building objects by combining smaller, more specialized components.
In JavaScript, composition can be achieved through object composition or functional composition.
Let's take a look at an example of object composition:
const canBark = {
bark() {
console.log("Woof!");
}
};
const canSwim = {
swim() {
console.log("Swimming...");
}
};
const dog = Object.assign({}, canBark, canSwim);
dog.bark(); // Output: "Woof!"
dog.swim(); // Output: "Swimming..."
In this example, we have two behavior objects, canBark and canSwim, which define the bark() and swim() methods, respectively. By using Object.assign(), we combine these behavior objects into a new object called dog. The dog object now has both the bark() and swim() methods, effectively inheriting their behaviors.
Functional composition is another approach that involves combining functions to create more complex behaviors. It can be achieved using higher-order functions or function composition utilities like compose() or pipe().
const add = (a, b) => a + b;
const multiply = (a, b) => a * b;
const addAndMultiply = (a, b, c) => multiply(add(a, b), c);
console.log(addAndMultiply(2, 3, 4)); // Output: 20
In this example, we define two simple functions, add() and multiply(). The addAndMultiply() function composes these two functions to perform addition and multiplication in a single step, resulting in a cleaner and more concise code.
By leveraging composition, we can create modular and reusable code by combining smaller components to form more complex objects or behaviors.
Conclusion
Encapsulation, abstraction, and composition are crucial concepts in object-oriented programming that promote code organization, maintainability, and reusability. In JavaScript, these principles can be effectively applied using classes, access modifiers, abstract methods, object composition, or functional composition techniques.
By following these principles, you can create cleaner, more maintainable, and more flexible code, enabling you to build robust and scalable JavaScript applications.
Remember, practice and experimentation are key to mastering these concepts, so don't hesitate to apply them in your own projects and explore their possibilities.
HAPPY CODING!!!
😎😎😎
If you like my post please consider hitting the donate button, so I can continue to write these articles.
Thank You
Great job, Ken! I've never delved this deep into object-oriented programming with regards to Composition, Abstraction, and Encapsulation. Thank you for taking the time to document and share your teachings. It's important for any developer to understand how to construct and develop concise and scalable code, as this ensures wider adoption and usage.