Higher Order Functions in JavaScript
- ken quiggins
- Jun 5, 2023
- 3 min read
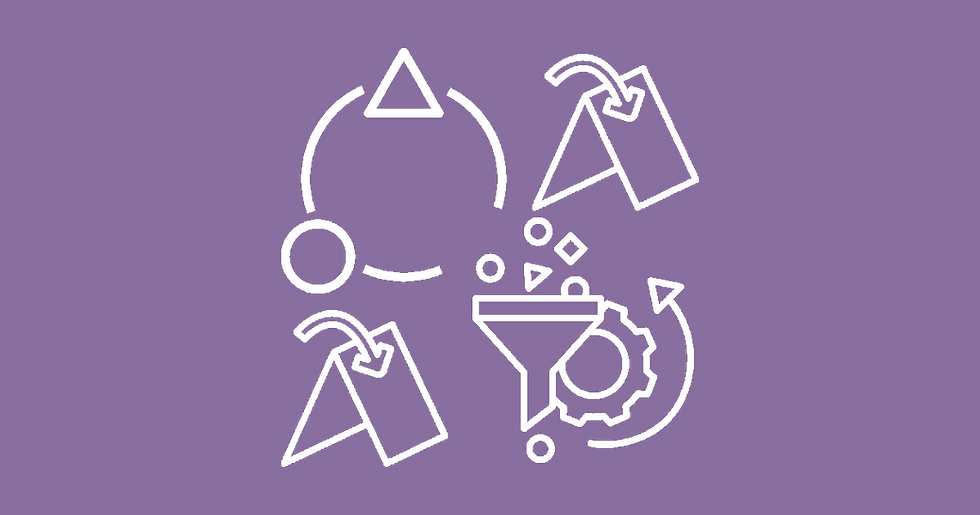
In JavaScript, functions are treated as first-class citizens, which means they can be assigned to variables, passed as arguments to other functions, and even returned from functions. This powerful feature allows us to use higher order functions, which are functions that take one or more functions as arguments or return a function as their result. Higher order functions are a fundamental concept in functional programming and can greatly enhance the expressiveness and flexibility of our code. In this article, we'll explore higher order functions in JavaScript and look at some examples of their practical uses.
1. Map
The map function is commonly used to transform each element of an array into a new value. It takes a callback function as an argument, which is executed for each element in the array. The map function then returns a new array containing the transformed values.
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = numbers.map((number) => number * number);
console.log(squaredNumbers); // [1, 4, 9, 16, 25]
2. Filter
The filter function is used to create a new array that contains only the elements that pass a certain condition. It also takes a callback function as an argument, which is applied to each element in the array. The filter function then returns a new array containing only the elements for which the callback function returns true.
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter((number) => number % 2 === 0);
console.log(evenNumbers); // [2, 4]
In this example, we use the filter function to extract only the even numbers from the numbers array. The callback function (number) => number % 2 === 0 checks if a number is divisible by 2, and if so, returns true. The filter function creates a new array containing only the numbers for which the callback function returns true.
3. Reduce
The reduce function is used to accumulate a single value by iterating over an array. It takes a callback function and an initial value as arguments. The callback function receives two parameters: the accumulated value and the current element of the array. The callback function performs an operation using these two values and returns the updated accumulated value. The reduce function applies the callback function to each element of the array, updating the accumulated value along the way.
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((accumulator, number) => accumulator + number, 0);
console.log(sum); // 15
In this example, we use the reduce function to calculate the sum of all numbers in the numbers array. The callback function (accumulator, number) => accumulator + number adds the current number to the accumulated sum and returns the updated sum. The reduce function applies this callback to each element of the array, starting with an initial sum value of 0.
Conclusion
Higher order functions like map, filter, and reduce provide powerful tools for working with arrays in JavaScript. They enable us to write more concise and expressive code by abstracting away common iteration and transformation patterns. By understanding and utilizing higher order functions, we can make our code more functional, modular, and easier to reason about.
In this article, we explored the map, filter, and reduce functions and demonstrated their practical uses. However, JavaScript offers many more higher order functions, each with its own specific purpose. By mastering these functions, you can unlock the full potential of functional programming in JavaScript and improve the quality of your code.
Remember, practice makes perfect! So go ahead and experiment with higher order functions in your own projects to gain a deeper understanding of their capabilities.
Happy coding!
Comments