Effortlessly Convert SVGs to React Components
- ken quiggins
- May 4, 2023
- 2 min read
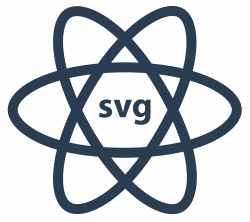
In this blog post, we will walk through the process of converting an SVG file into a React component using the SVGR tool, and then integrating the component into a navigation bar. This method is particularly useful for including custom logos or icons in your project without the need for additional libraries.
Step 1: Converting SVG to React Component
First, we'll use the SVGR tool to convert our SVG file into a React component. You can access the SVGR tool here.
Open the tool and paste your SVG code into the left side of the page.
The output on the right side will show the React component code. You can adjust the settings to your liking, such as changing the size or optimizing the code.
Copy the generated React component code.
In our example, we've changed the width and height from 800x800 to 50x50:
const SvgComponent = (props) => (
// ... rest of the component code ...
<svg
xmlns="http://www.w3.org/2000/svg"
xmlSpace="preserve"
width={50}
height={50}
viewBox="0 0 1000 1000"
{...props}
>
// ... rest of the component code ...
)
export default SvgComponent
Step 2: Creating the Logo Component
Next, we'll create a new folder named logo in our components folder, and add a file named logo.component.jsx. Paste the output from the SVGR tool into this file.
Step 3: Importing the Logo Component
In the navigation.component.jsx file, we'll import the newly created logo component:
// depending on your folder structure your import may be different
import SvgComponent from '../../components/logo/logo.component';
Step 4: Adding the Logo Component to the Navigation Bar
Now that we have the logo component imported, we can add it to our navigation bar. Update the Navigation component in the navigation.component.jsx file:
const Navigation = () => {
return (
<Fragment>
<div className="navigation">
<Link className="logo-container" to='/'>
<SvgComponent />
</Link>
<div className="nav-links-container">
<Link to="/shop" className="nav-link">
SHOP
</Link>
</div>
</div>
<Outlet />
</Fragment>
)
};
export default Navigation;
And that's it! We have successfully converted an SVG file into a React component using the SVGR tool and integrated it into our navigation bar. This approach makes it easy to include custom logos or icons in your React projects without the need for additional libraries.
Komentarze